Contents of this blog:
- Python lists.
- Built-in list functions.
- Accept list elements from user.
- List comprehension.
- List concatenation.
A variable can store only one value at a time, but lists are built in data types which can store multiple values.
a=[1,2,3]
1,2 and 3 are list items. They have different positions in the list called index. Indexing start from 0. Which means 1 has index 0, 2 has index 1 and so on.
Python lists are changeable, we can add or remove values from a list and can merge two lists.
Below are some built in list functions:-
a.append(value) | Adds elements at the end of the list. |
a.count(value) | returns the number of times the given value is repeated in the list. |
a.extend(list) | add given list to the end of original list. |
a.insert(index,value) | adds the element in the given position. |
a.pop(index) | remove the element at given position and also prints the removed element if printed. |
a.reverse() | reverse the list |
a.remove(value) | removes the value from the list |
a.copy() | make a copy of the original list |
len(list name) | gives the length of the list. |
a.index(value) | gives the index of the given value and for repeated values,first index containing the value is given. |
a.sort() | sort the list ascending order. |
Example:-
a=[1,3,4,5,6]
a.append(9)
print("new array after appending",a)
b=[4,7,8]
a.extend(b)
print("a after merging b",a)
a.insert(3,6)
print("new array after insertion",a)
print("no. of 6 in a is ",a.count(6))
print("position of 4 is",a.index(4))
print("the removed element",a.pop(4))
print("a after the element is removed",a)
a.reverse()
print("reverse",a)
b=a.copy()
print("copy of a",b)
a.sort()
print("after sorting a",a)
print("length of the current list",len(a))
a.remove(6)
print("a after removing 6",a)
Output:-

Accepting list elements from user:-
Step1: Initialise array . a=[]
Step2: Run a loop from 0 to the size of list you want
Step3: write the input function and append the value within the loop
example:-
a=[]
print("enter the number")
for i in range(0,3):
element=int(input())
a.append(element)
print(a)
Output:-

Now a question on how to work with the elements of a list.
Question:- Accept 4 numbers in a list and print the list. Display the greatest elements of the list.
a=[]
max=0
for i in range(0,4):
ele=int(input())
a.append(ele)
print("the list is ",a)
for i in range(0,4):
if a[i]>max:
max=a[i]
print("the greatest number is ",max)
Output:-

We have initialised max=0. Inside the loop we are checking if the ith element (denoted by a[i]) is greater than the current max. If it is that a[i] element becomes the max.
like the 0th element is 45 , it’s greater than max(which is initialised to 0) ,so it becomes new max. The next element 62 is greater than 45 , 62 becomes new max. Now 3 is less than 62, 62 remains max. Now 89 is greater than 62, 89 is max and is printed outside the loop.
Question:- Accept elements of a list and print the matrix. If the elements are even, square them , keep the odd elements as it is.
a=[]
for i in range(0,6):
ele=int(input())
a.append(ele)
print("the list is ",a)
for i in range(0,6):
if a[i]%2==0:
a[i]=a[i]**2
print("the new list",a)
OUTPUT:-

In the loop , we are checking the elements in the ith position of the list denoted by a[i] and if they are even, we replacing a[i] by a[i]**2.
Question:- Accept elements of a list and print it. Now make another list by taking only the odd numbers from previous list.
a=[]
ls=[]
for i in range(0,6):
ele=int(input())
a.append(ele)
print("the list is ",a)
for i in range(0,6):
if a[i]%2!=0:
ls.append(a[i])
print("the new list ",ls)
Output:-

Its like the previous program, but checking the odd number and only appending the odd numbers to an empty list ls[].
Now this programme can be done more efficiently using list comprehension.
List comprehension:- An efficient way to do the same operation to the elements of the list .
Syntax:-
[expre for
element in
iterable if
condition]
where:- expre= expression(the operation we want to perform like x**2)
iterable= any iterable eg: list, tuple,range()
elements= elements of the list
eg: lst=[x for x in a]
So let’s do the previous program by list comprehension.
a=[]
for i in range(0,6):
ele=int(input())
a.append(ele)
print("the list is ",a)
ls=[x for x in a if x%2!=0]
print(ls)
Output will also be the same .
Question:- Make a list of square of numbers divisible by 7 from 1 to 50.
lst=[x**2 for x in range(1,51) if x%7==0]
print(lst)
OUTPUT:-

Question:- multiply each element of the list with 3.
a=[3,4,8,5,1,9]
lst=[2*x for x in a]
print(lst)
Output:-
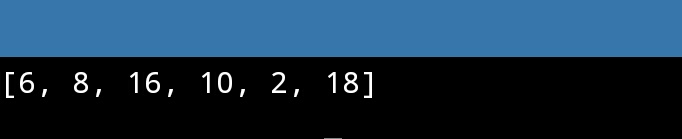
Notice that list comprehension extraction is nothing but compact way of writing for loop.
a=[3,4,8,5,1,9]
lst=[2*x for x in a]
which can be written as,
a=[3,4,8,5,1,9]
lst=[]
for x in a:
x=2*x
lst.append(x)
print(a)
Notice the colour coded portions, it will be helpful in understanding the complex list comprehensions.
List Concatenation :- It means addition of two lists.
It can achieved by many methods:
a)) By using array.extend() :- syntax and examples given above
b)) By using + operator :-
a=[3,4,8,5,1,9]
lst=[7,8,6,98,43,21]
print(a+lst)
Output:-

c)) By using list comprehension:-
syntax for joing two list:
lst=[n for
m in
[list1,list2] for
n in
m]
a=[3,4,8,5,1,9]
lst1=[7,8,6,98,43,21]
lst=[n for m in [a,lst1] for n in m]
print("the merged list is ")
print(lst)

Those who want to understand how the syntax is working, lets write it same way using for loop.
a=[3,4,8,5,1,9]
lst1=[7,8,6,98,43,21]
lst=[]
for m in [a,lst1]:
for n in m:
lst.append(n)
print(lst)
values of m is a and lst1 that is [3,4,8,5,1,9] and [7,8,6,98,43,21] and n runs between the values of m that is it picks up the values of individual lists .
m | n |
1st value of m [3,4,8,5,1,9] | for n in m so values of n will be 3,4,8,5,1,9 |
2nd value of m [7,8,6,98,43,21] | and second time values of n 7,8,6,98,43,21 |
So the values of n are printed within the new list . So the the previous lists are merged.