Contents of this blog:
- Python program to find sum, difference and product.
- Program to find area and perimeter of rectangle.
- Decision making in python.
- if statement
- if else statement
- nested if statement
- Program to accept three numbers. If they are unequal,display the greatest number.
- Program to accept a number and if it’s an even number, multiply the number by two if the number is less than 10 , multiply the number by 3 if it’s equal to 10 and multiply by 4 if it’s greater than 10.
- Try and except block.
Getting started with python programming:-
Let’s start by writing some simple program.
Q:- Write a program to find sum, difference and product of two numbers(take input).
a=int(input(" enter a"))
b=int(input(" enter b"))
sum=a+b
dif=a-b
pro=a*b
print(" sum= ",sum)
print(" diff= ",dif)
print(" product= ",pro)
Output:-
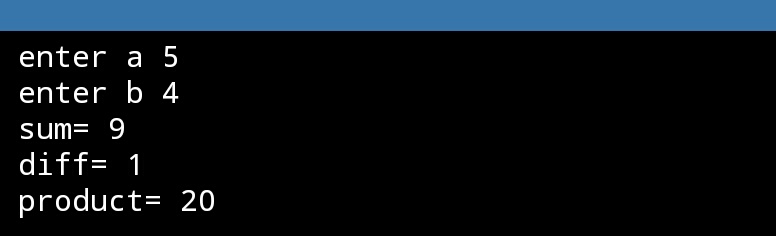
Q:- Write a program find area and perimeter of a rectangle.( Take input the sides)
a=float(input(" side1= "))
b=float(input(" side2= "))
area=a*b
perimeter=2*(a+b)
print(" area= ",area)
print(" perimeter= ", perimeter)
Output:-
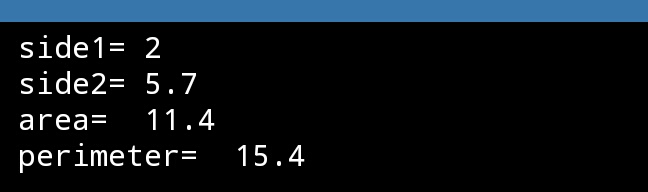
Decision making in python:-
The previous programs were simple, but many programs require complex logic which need us to specify some type of conditions and some operations are performed based on that. If this then that…if a is less than 100 , print a. These things can be achieved by following control statements, given below.
Point to be noted, < > in the given syntax is the indentation block. In python , you must indent equal amount of white space(preferably tabs) under every if statement. It is used to indicate a block of code. Python will give error if you skip indentation.
a. if statement
syntax:-
if condition:
< >statement

b. if-elif-else statement.
syntax:-
if condition1:
< >statement 1
elif condition2:
< >statement2
else:
< >statement
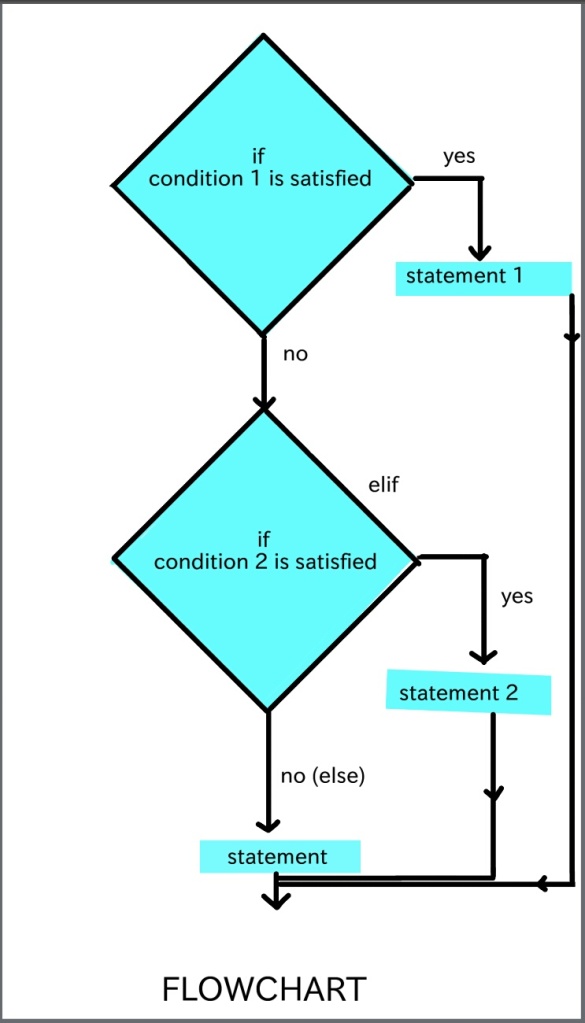
c. nested if statement syntax
if condition1:
< >if condition 2:
< >< >statement 1
< >else:
< >< >statement 2
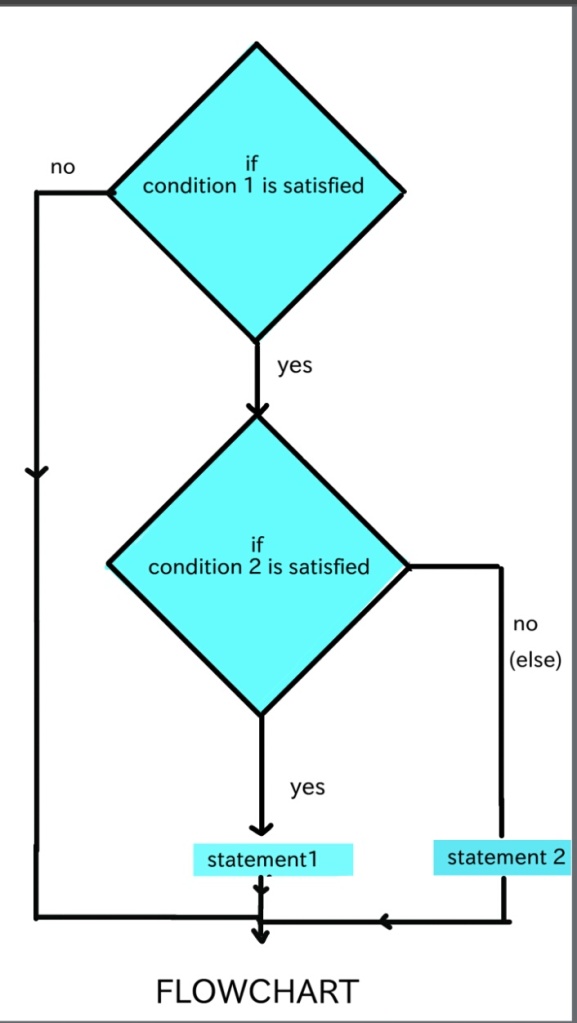
Now, some programs based on decision making.
Question:- Write a program to accept three numbers. If they are unequal,display the greatest number. If they are equal, display they are equal.
print("enter three numbers")
a=int(input())
b=int(input())
c=int(input())
if a<b and c<b:
print("greatest number is ",b)
elif b<a and c<a:
print("greatest number is ",a)
elif b<c and a<c:
print("greatest number is ",c)
else:
print("all are equal")
Output1:-
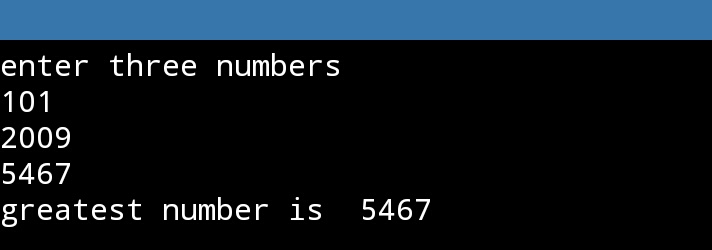
Output2:-

Question 2:– Write a program to accept a number and if it’s an even number, multiply the number by two if the number is less than 10 , multiply the number by 3 if it’s equal to 10 and multiply by 4 if it’s greater than 10.
a=int(input("enter= "))
if a%2==0:
if a<10:
print(a*2)
if a==10:
print(a*3)
if a>10:
print(a*4)
else:
print("odd")
Output:-
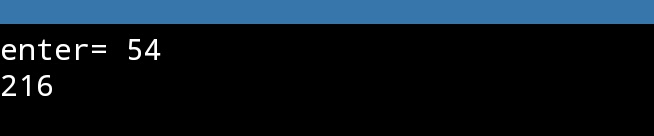
Check other outputs.
Try and except block:- This block is used to catch any error in code and give some message to the programmer.
syntax
try:
< >statement or code
except:
< >print(“error”)
That try block tries catches error in the piece of code and if any error occurs the message in the except block is delivered.
example:
try:
if(x<10): #here x is not defined, an error expected.
print("yes")
except:
print("an error has occured")
Output
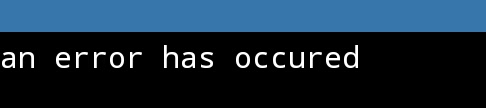